hildon.RangeEditor
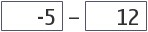
Used to set a number range from specific limits.
Description
hildon.RangeEditor
is used to set a number range/interval from specific limits.
Ancestry
Up to the first non-hildon ancestor:
... +-- gtk.Container +-- hildon.RangeEditor
Usage Example
import gtk import hildon window = hildon.Window() window.set_title("Test App") range_editor = hildon.RangeEditor() range_editor.set_limits(-100, 100) range_editor.set_range(-10, 23) range_editor.set_separator("to") window.add(range_editor) window.show_all() gtk.main()
Properties
Name | Access | Description |
---|---|---|
"min" |
Read/Write/Construct | Minimum value in a range. |
"max" |
Read/Write/Construct | Maximum value in a range. |
"lower" |
Read/Write/Construct | Current value in the entry presenting lower end of selected range. |
"higher" |
Read/Write/Construct | Current value in the entry presenting higher end of selected range. |
"separator" |
Read/Write/Construct | Separator string to separate lower and higher entries. |
Style Properties
Name | Access | Description |
---|---|---|
"hildon_range_editor_entry_alignment" |
Read | Hildon RangeEditor entry alignment. |
"hildon_range_editor_separator_padding" |
Read | Hildon RangeEditor separaror padding. |
Constructor
hildon.RangeEditor
contains two gtk.Entry
that accepts numbers and minus.
hildon.RangeEditor()
|
|
---|---|
Returns | A new hildon.RangeEditor widget. |
Methods
get_range
Gets the current range (the lower and higher entry values).
hildon.RangeEditor.get_range()
|
|
---|---|
Returns | A tuple of the form (lower, higher) containing the current range set. |
set_range
Sets the current range (the lower and higher entry values).
hildon.RangeEditor.set_range(lower, higher)
|
|
---|---|
lower |
Interval's lower bound. |
higher |
Interval's upper bound. |
set_limits
Sets the limits for allowable range values. The range will be forced to stay within these limits.
hildon.RangeEditor.set_limits(min, max)
|
|
---|---|
min |
Minimum allowable lower bound. |
max |
Maximum allowable upper bound. |
get_lower
Gets range's lower entry value.
hildon.RangeEditor.get_lower()
|
|
---|---|
Returns | Interval's lower bound. |
set_lower
Sets range's lower entry value.
hildon.RangeEditor.set_lower(value)
|
|
---|---|
value |
Interval's lower bound. |
get_higher
Gets range's higher entry value.
hildon.RangeEditor.get_higher()
|
|
---|---|
Returns | Interval's upper bound. |
set_higher
Sets range's higher entry value.
hildon.RangeEditor.set_higher(value)
|
|
---|---|
value |
Interval's upper bound. |
get_min
Gets the minimun allowable value for range's lower entry.
hildon.RangeEditor.get_min()
|
|
---|---|
Returns | Minimum allowable lower bound. |
set_min
Sets the minimun allowable value for range's lower entry.
hildon.RangeEditor.Set_min(value)
|
|
---|---|
value |
Minimum allowable lower bound. |
get_max
Gets the maximun allowable value for range's higher entry.
hildon.RangeEditor.get_max()
|
|
---|---|
Returns | Maximum allowable upper bound. |
set_max
Sets the maximun allowable value for range's higher entry.
hildon.RangeEditor.Set_max(value)
|
|
---|---|
value |
Maximum allowable upper bound. |
get_separator
Gets the string that separates lower and higher entries.
hildon.RangeEditor.get_separator()
|
|
---|---|
Returns | String that separates lower and higher entries. |
set_separator
Sets the string that separates lower and higher entries.
hildon.RangeEditor.set_separator(separator)
|
|
---|---|
separator |
String that separates lower and higher entries. |
Improve this page