hildon.Seekbar
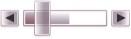
hildon.Seekbar
is used to seek a place from a content. Can be used eg. to browse a mp3 track.
Description
hildon.Seekbar
is used to seek a place from a content. Can be used eg. to browse a mp3 track.
Ancestry
Up to the first non-hildon ancestor:
... +-- gtk.Scale +-- hildon.Seekbar
Usage Example
import gobject import gtk import hildon def increase_fraction(seekbar): if seekbar.get_fraction() + 1 < seekbar.get_total_time(): seekbar.set_fraction(seekbar.get_fraction() + 1) result = True else: seekbar.set_fraction(seekbar.get_total_time()) result = False seekbar.notify("fraction") seekbar.queue_draw() return result; def control_changed(widget, label): label.set_text("Position: %s | Fraction: %s | Total time: %s" % (widget.get_position(), widget.get_fraction(), widget.get_total_time())) def fraction_changed(widget, param_spec, label): control_changed(widget, label) window = hildon.Window() window.set_title("Test App") label = gtk.Label() seekbar = hildon.Seekbar() seekbar.set_total_time(180) seekbar.set_fraction(10) seekbar.set_position(0) seekbar.connect("value-changed", control_changed, label) seekbar.connect("notify::fraction", fraction_changed, label) control_changed(seekbar, label) #Init label text vbox = gtk.VBox(False, 10) vbox.pack_start (seekbar) vbox.pack_start (label) window.add(vbox) gobject.timeout_add(500, increase_fraction, seekbar) window.show_all() gtk.main()
Constructor
Creates a new hildon.Seekbar
widget.
hildon.Seekbar()
|
|
---|---|
Returns | A new hildon.Seekbar widget. |
Methods
get_total_time
Gets the total playing time of stream in seconds.
hildon.Seekbar.get_total_time()
|
|
---|---|
Returns | The total time. |
set_total_time
Sets the total playing time of stream in seconds.
hildon.Seekbar.set_total_time(time)
|
|
---|---|
time |
The total time. |
get_position
Gets the current position in stream in seconds.
hildon.Seekbar.get_position()
|
|
---|---|
Returns | The current position in stream. |
set_position
Sets the current position in stream in seconds.
hildon.Seekbar.set_position(time)
|
|
---|---|
time |
The current position in stream. |
get_fraction
Gets current fraction related to the progress indicator. It must be between zero and seekbar's total time.
hildon.Seekbar.get_fraction()
|
|
---|---|
Returns | The current fraction related to the progress indicator. |
set_fraction
Sets current fraction related to the progress indicator. It must be between zero and seekbar's total time.
hildon.Seekbar.set_fraction(fraction)
|
|
---|---|
fraction |
The current fraction related to the progress indicator. |
Improve this page