hildon.GetPasswordDialog
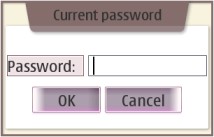
hildon.GetPasswordDialog
is used to get a password.
Description
hildon.GetPasswordDialog
is used to get a password.
Ancestry
Up to the first non-hildon ancestor:
... +-- gtk.Dialog +-- hildon.GetPasswordDialog
Usage Example
import gtk import hildon def get_password(widget, label, passwordDialog): passwordDialog.set_property ("password", "") #Clear the password string, in case it was already set. response = passwordDialog.run() passwordDialog.hide() if response == gtk.RESPONSE_OK: label.set_text("Password typed is: %s" % passwordDialog.get_password()) window = hildon.Window() window.set_title("Test App") label = gtk.Label("No password entered.") passwordDialog = hildon.GetPasswordDialog(window, True) button = gtk.Button("Click and type a password") button.connect("clicked", get_password, label, passwordDialog) vbox = gtk.VBox(False, 10) vbox.pack_start (button) vbox.pack_start (label) window.add(vbox) window.show_all() gtk.main()
Properties
Name | Access | Description |
---|---|---|
"domain" |
Read/Write | Set domain (content) for optional label. |
"password" |
Read/Write | Set content for password entry. |
"numbers_only" |
Read/Write | Set entry to accept only numeric values. |
"caption-label" |
Read/Write | The text to be set as the caption label. |
"max-characters" |
Read/Write | The maximum number of characters the password dialog accepts. |
"get-old" |
Read/Write/Construct Only | True if dialog is a get old password dialog, False if dialog is a get password dialog. |
Constructor
Creates a new hildon.GetPasswordDialog
widget.
hildon.GetPasswordDialog(parent, get_old_password_title)
|
|
---|---|
parent |
Its parent window. |
get_old_password_title |
False creates a "get new password" dialog and True creates a "get current password" dialog. |
Returns | A new hildon.GetPasswordDialog widget. |
Methods
set_domain
Sets the optional descriptive text.
hildon.GetPasswordDialog.set_domain(domain)
|
|
---|---|
domain |
The domain or some other descriptive text to be set. |
set_title
Sets the dialog title.
hildon.GetPasswordDialog.set_title(new_title)
|
|
---|---|
new_title |
The new dialog title. |
set_caption
Sets the text that goes besides the password field. e.g.: "Password:" .
hildon.GetPasswordDialog.set_caption(new_caption)
|
|
---|---|
new_caption |
The new dialog caption. |
set_max_characters
Sets the maximum number of characters the password dialog accepts.
hildon.GetPasswordDialog.set_max_characters(max_characters)
|
|
---|---|
max_characters |
The maximum number of characters the password dialog accepts. |
get_password
Gets the password entered in the dialog.
hildon.GetPasswordDialog.get_password()
|
|
---|---|
Returns | The password entered in the dialog (or the default if one hasn't been entered yet). |
Improve this page